LISTS IN PYTHON
In this tutorial, we are going to learn about lists in python and how to perform indexing, splitting, updating items in a list, adding elements to the list and iterating through the list.
What are Lists in Python?
Python list is used to store the sequence of different data types. Python, however, includes six kinds of data types that can store the sequences, but list is the most prevalent and reliable form.
A list may be defined as a collection of different types of values or items. The items in the list are divided by the comma (,) and items are encapsulated inside the square brackets [].
You can define lists as follows:
names = ["Hira", 302, "Paris"]
numbers = [1, 2, 3, 4, 5, 6]
random = [3, "John"]
print(names)
print(numbers)
print(random)
Indexing and Splitting in Lists
Indexing is processed in the same manner as the strings are processed. Using the slice operator [], the list components can be accessed. The index begins at 0 and ends at -1. The list’s first component is placed in the 0th index, the list’s second component is placed in the 1st index, and so on.
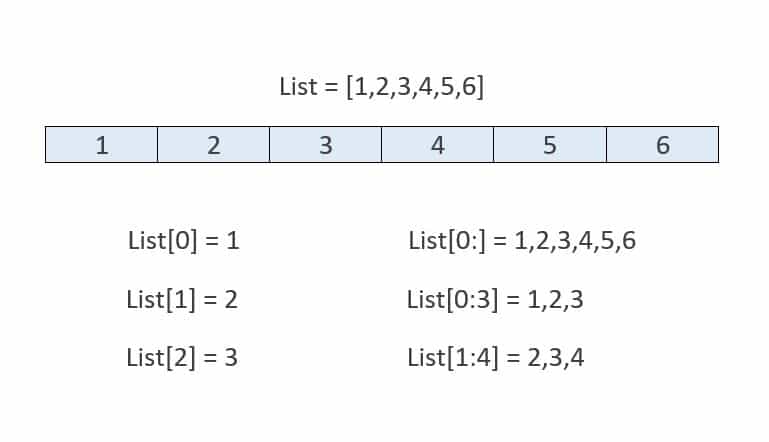
lists
Python gives us the flexibility to use negative indexing as well, unlike other languages. From the right, negative indexes are counted. The list’s last component (most right) has the index-1, its next element is placed in the index-2 (from right to left) and so on until most of the left component is found.
Updating an Item in List
Lists are mutable, meaning that unlike string or tuple, their elements can be changed. To alter an item or a variety of items, we can use assignment operator (=). For Example:
string = ['p','y','t','h','o','n']
del string[2]
print(string)
Also, Python provides us with the remove() method or a pop() method if we don’t know which component to remove from the list. The pop() method removes the last element of the list if the index is not provided.
string = ['p','y','t','h','o','n']
string.remove('p')
string.pop()
print(string)
Adding elements to the list
You can add new elements to an existing list by using the append() method. The only condition with append() method is that you can only append or add new elements to the end of the list.
fruits = ['apple', 'banana', 'orange', 'kiwi']
fruits.append('grapes')
print(fruits)
Iterating a List
You can iterate through a list as well by using a for loop
For more reference, click here
numbers = [1,2,3,4,5,6,7,8]
for n in numbers:
print(n)